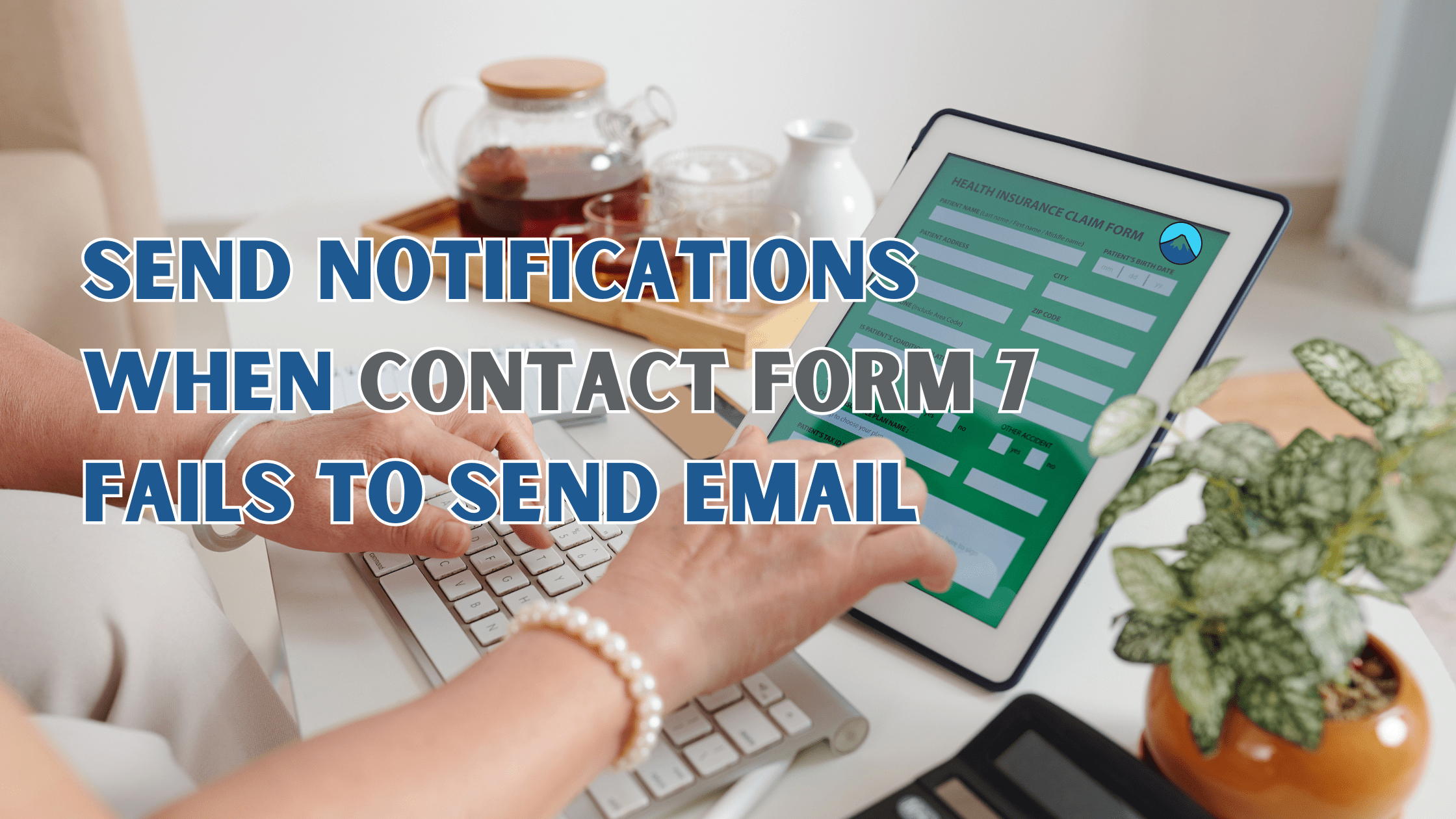
How to Send Notifications via PostSMTP When Contact Form 7 Fails to Send an Email
制作・開発Contact Form 7 (CF7) is one of the most widely used WordPress plugins for creating contact forms. However, there are times when emails sent via CF7 may fail due to various reasons like mail server issues or misconfigurations. In such cases, it is important to be notified so that we can take timely action.
By default, CF7 doesn’t provide any direct notification when an email fails to send. Fortunately, if we’re using PostSMTP to handle email sending, we can hook into CF7’s failure events and send an email notification using PostSMTP itself. In this guide, I’ll show how to set up a custom function to notify whenever Contact Form 7 fails to send an email using PostSMTP’s email service.
Why Use PostSMTP for Notification?
While WordPress has its built-in wp_mail()
function to send emails, PostSMTP is a more robust solution that offers better reliability and reporting for email delivery. It integrates with various third-party email services such as Gmail, SendGrid, or any SMTP server, making it more versatile for handling email notifications.
Steps to Send Notifications via PostSMTP
Below is a step-by-step guide to implementing this functionality, along with the updated code that ensures a notification email is sent when CF7 fails to send one.
Step 1: Hook Into Contact Form 7 Email Failure
WordPress provides an action hook wpcf7_mail_failed
that is triggered whenever Contact Form 7 fails to send an email. We can execute a custom function to send a notification email by hooking into this event.
Step 2: Use PostSMTP’s Mail Functionality
Instead of using the default wp_mail()
, we’ll use the PostmanWpMail
class provided by PostSMTP to send the email notification. This ensures the email notification will be sent using the same reliable method as other emails sent through PostSMTP.
Step 3: Implement the Custom Function
Here’s the updated code to achieve the desired functionality:
add_action('wpcf7_mail_failed', 'send_email_using_post_smtp_on_cf7_failure', 10, 1);
function send_email_using_post_smtp_on_cf7_failure($contact_form) {
// Set the recipient address
$recipient_email = 'admin@email.com'; // Replace with email address
$subject = 'Contact Form 7 Failed to Send Email from ' . get_bloginfo('name');
$message = 'A Contact Form 7 submission failed to send an email. Please check the system for issues.';
// Prepare the email data
$email_data = array(
'to' => $recipient_email,
'subject' => $subject,
'body' => $message,
'headers' => array('Content-Type: text/html; charset=UTF-8'),
);
// Use PostSMTP's email sending mechanism
$mailer = new PostmanWpMail();
$mailer->send($email_data['to'], $email_data['subject'], $email_data['body'], $email_data['headers']);
}
Explanation of the Code
- Hooking into CF7 Failure: The
add_action()
function hooks intowpcf7_mail_failed
. This action is triggered whenever CF7 fails to send an email. - Recipient Email: The
$recipient_email
variable should be replaced with our email address where we want to receive failure notifications. - Email Subject and Body: The email’s subject and message body are defined with variables like
$subject
and$message
. We can customize the message to include more details if needed. Here, we append the site’s name to the email subject usingget_bloginfo('name')
. - PostSMTP Integration: Instead of using WordPress’s default
wp_mail()
function, we utilize thePostmanWpMail
class from PostSMTP. The method$mailer->send()
is used to send the email via PostSMTP’s configured service.
Step 4: Ensure PostSMTP Is Installed and Active
Before using this solution, make sure that the PostSMTP plugin is installed and active on our WordPress site. This plugin is responsible for sending emails and must be correctly configured with our SMTP or third-party email service (like Gmail, SendGrid, etc.).
Benefits of This Approach
- Reliability: PostSMTP is a powerful tool for ensuring that our emails are sent reliably via third-party SMTP services.
- Error Logging: PostSMTP also offers great logging features, so we can easily troubleshoot any email issues.
- Immediate Notification: We’ll be notified as soon as an email fails, which helps in faster response times to correct any issues.
With just a few lines of code, we can enhance our website’s email reliability by ensuring we are notified when a Contact Form 7 email fails to send. By leveraging the power of PostSMTP, we can avoid using the default wp_mail()
and instead rely on a more robust email solution.
Make sure to test this solution in our development environment before deploying it to our live site!